Gold nano-sphere on substrate¶
!!Attention!!: pyGDM2-retard is still in beta version and is to be used with caution.
Example author: Peter Wiecha (retarded propagator by G. Colas des Francs)
In this example, we calculate the extinction spectra of a gold sphere deposited on a dielectric substrate as well as on a gold film. For comparison, an extinction spectrum of the sphere on a gold substrate can be found in Patoux et al. [1]. The script requires the extension package pyGDM2-retard (https://pypi.org/project/pyGDM2-retard/). It can be installed via pip:
pip install -U pyGDM2-retard
[1]: Patoux et al. Polarizabilities of complex individual dielectric or plasmonic nanostructures. PRB 101, 235418 (2020). (https://link.aps.org/doi/10.1103/PhysRevB.101.235418)
[1]:
import numpy as np
import matplotlib.pyplot as plt
from pyGDM2 import structures
from pyGDM2 import materials
from pyGDM2 import fields
from pyGDM2 import core
from pyGDM2 import propagators
from pyGDM2 import linear
from pyGDM2 import tools
## the external package for the retarded Green's Dyads
from pyGDM2_retard import propagators_retarded
setup several simulations¶
[2]:
## --- structure: gold nano-sphere
step = 12 # for the paper we used a step of 6.67nm
mesh = 'hex'
material = materials.gold()
geo_small = structures.sphere(step, R=25/step, mesh=mesh)
struct_small = structures.struct(step, geo_small, material)
geo_large = structures.sphere(step, R=50/step, mesh=mesh)
struct_large = structures.struct(step, geo_large, material)
## --- incident field: plane wave from top, lin. pol. along X
field_generator = fields.plane_wave
wavelengths = np.linspace(450,700,31)
kwargs = dict(inc_angle=180, E_p=1.0, E_s=0.0)
efield = fields.efield(field_generator,
wavelengths=wavelengths, kwargs=kwargs)
## --- define environments
dyads_glass_quasistatic = propagators.DyadsQuasistatic123(n1=1.5, n2=1.5, n3=1, spacing=0)
dyads_glass_retard = propagators_retarded.DyadsRetard123(n1=1.5, n2=1.5, n3=1, spacing=0)
dyads_goldfilm_quasistatic = propagators.DyadsQuasistatic123(n1=1.5, n2=materials.gold(), n3=1, spacing=50)
dyads_goldfilm_retard = propagators_retarded.DyadsRetard123(n1=1.5, n2=materials.gold(), n3=1, spacing=50)
## --- all configurations to simulate
conf_list = [
## small sphere
dict(
dyads=dyads_glass_quasistatic,
struct=struct_small,
title='R=25nm, on glass - quasistatic'
),
dict(
dyads=dyads_glass_retard,
struct=struct_small,
title='R=25nm, on glass - with retard'
),
dict(
dyads=dyads_goldfilm_quasistatic,
struct=struct_small + [0,0,50], # shift on top of gold film
title='R=25nm, gold film - quasistatic'
),
dict(
dyads=dyads_goldfilm_retard,
struct=struct_small + [0,0,50], # shift on top of gold film
title='R=25nm, gold film - with retard'
),
## large sphere
dict(
dyads=dyads_glass_quasistatic,
struct=struct_large,
title='R=50nm, on glass - quasistatic'
),
dict(
dyads=dyads_glass_retard,
struct=struct_large,
title='R=50nm, on glass - with retard'
),
dict(
dyads=dyads_goldfilm_quasistatic,
struct=struct_large + [0,0,50], # shift on top of gold film
title='R=50nm, gold film - quasistatic'
),
dict(
dyads=dyads_goldfilm_retard,
struct=struct_large + [0,0,50], # shift on top of gold film
title='R=50nm, gold film - with retard'
)
]
structure initialization - automatic mesh detection: hex
structure initialization - consistency check: 69/69 dipoles valid
structure initialization - automatic mesh detection: hex
structure initialization - consistency check: 515/515 dipoles valid
run the simulations¶
[3]:
all_spec = []
all_tit = []
for conf in conf_list:
## --- load config
all_tit.append(conf['title'])
struct = conf['struct']
dyads = conf['dyads']
## --- create and run the simulation
sim = core.simulation(struct, efield, dyads)
sim.scatter()
## -- extinction spectrum
wl, spec = tools.calculate_spectrum(sim, 0, linear.extinct)
ex,sc,ab = spec.T
all_spec.append(ex)
timing for wl=450.00nm - setup: EE 1543.3ms, inv.: 445.9ms, repropa.: 588.9ms (1 field configs), tot: 2578.4ms
timing for wl=458.33nm - setup: EE 4.4ms, inv.: 1.6ms, repropa.: 1.9ms (1 field configs), tot: 7.9ms
timing for wl=466.67nm - setup: EE 3.8ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 7.2ms
timing for wl=475.00nm - setup: EE 3.7ms, inv.: 1.5ms, repropa.: 1.9ms (1 field configs), tot: 7.1ms
timing for wl=483.33nm - setup: EE 3.7ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 7.0ms
timing for wl=491.67nm - setup: EE 3.7ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 7.1ms
timing for wl=500.00nm - setup: EE 3.7ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 7.0ms
timing for wl=508.33nm - setup: EE 3.6ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 6.9ms
timing for wl=516.67nm - setup: EE 3.5ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 6.8ms
timing for wl=525.00nm - setup: EE 3.4ms, inv.: 1.4ms, repropa.: 1.8ms (1 field configs), tot: 6.7ms
timing for wl=533.33nm - setup: EE 3.6ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 6.9ms
timing for wl=541.67nm - setup: EE 3.9ms, inv.: 1.5ms, repropa.: 1.9ms (1 field configs), tot: 7.3ms
timing for wl=550.00nm - setup: EE 3.4ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 6.7ms
timing for wl=558.33nm - setup: EE 3.4ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 6.8ms
timing for wl=566.67nm - setup: EE 3.7ms, inv.: 1.4ms, repropa.: 1.8ms (1 field configs), tot: 7.0ms
timing for wl=575.00nm - setup: EE 3.6ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 7.0ms
timing for wl=583.33nm - setup: EE 3.4ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 6.7ms
timing for wl=591.67nm - setup: EE 3.6ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 7.0ms
timing for wl=600.00nm - setup: EE 3.6ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 6.9ms
timing for wl=608.33nm - setup: EE 3.5ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 6.8ms
timing for wl=616.67nm - setup: EE 3.9ms, inv.: 1.4ms, repropa.: 2.0ms (1 field configs), tot: 7.4ms
timing for wl=625.00nm - setup: EE 3.4ms, inv.: 1.5ms, repropa.: 1.9ms (1 field configs), tot: 6.9ms
timing for wl=633.33nm - setup: EE 3.4ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 6.8ms
timing for wl=641.67nm - setup: EE 3.5ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 6.9ms
timing for wl=650.00nm - setup: EE 3.3ms, inv.: 1.5ms, repropa.: 1.9ms (1 field configs), tot: 6.7ms
timing for wl=658.33nm - setup: EE 3.5ms, inv.: 1.6ms, repropa.: 1.9ms (1 field configs), tot: 7.1ms
timing for wl=666.67nm - setup: EE 3.9ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 7.3ms
timing for wl=675.00nm - setup: EE 3.4ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 6.8ms
timing for wl=683.33nm - setup: EE 3.5ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 6.8ms
timing for wl=691.67nm - setup: EE 3.9ms, inv.: 1.5ms, repropa.: 1.9ms (1 field configs), tot: 7.4ms
timing for wl=700.00nm - setup: EE 3.7ms, inv.: 1.5ms, repropa.: 2.0ms (1 field configs), tot: 7.3ms
identification of identical tensors: 0.49s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.10s
timing for wl=450.00nm - setup: EE 844.6ms, inv.: 1.9ms, repropa.: 2.1ms (1 field configs), tot: 848.6ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.21s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=458.33nm - setup: EE 231.5ms, inv.: 1.7ms, repropa.: 2.2ms (1 field configs), tot: 235.4ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=466.67nm - setup: EE 231.3ms, inv.: 1.7ms, repropa.: 2.3ms (1 field configs), tot: 235.4ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=475.00nm - setup: EE 239.9ms, inv.: 1.8ms, repropa.: 3.2ms (1 field configs), tot: 245.0ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=483.33nm - setup: EE 240.0ms, inv.: 1.7ms, repropa.: 2.3ms (1 field configs), tot: 244.1ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=491.67nm - setup: EE 235.9ms, inv.: 1.8ms, repropa.: 2.2ms (1 field configs), tot: 240.0ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.23s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=500.00nm - setup: EE 244.0ms, inv.: 1.7ms, repropa.: 2.2ms (1 field configs), tot: 248.0ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=508.33nm - setup: EE 239.9ms, inv.: 1.8ms, repropa.: 2.2ms (1 field configs), tot: 244.1ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.23s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=516.67nm - setup: EE 241.5ms, inv.: 1.7ms, repropa.: 2.9ms (1 field configs), tot: 246.3ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=525.00nm - setup: EE 239.7ms, inv.: 1.8ms, repropa.: 2.3ms (1 field configs), tot: 243.9ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=533.33nm - setup: EE 237.5ms, inv.: 1.7ms, repropa.: 2.2ms (1 field configs), tot: 241.5ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.23s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=541.67nm - setup: EE 248.5ms, inv.: 1.8ms, repropa.: 3.0ms (1 field configs), tot: 253.3ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.23s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=550.00nm - setup: EE 244.7ms, inv.: 1.7ms, repropa.: 2.2ms (1 field configs), tot: 248.7ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=558.33nm - setup: EE 240.4ms, inv.: 1.9ms, repropa.: 2.2ms (1 field configs), tot: 244.6ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.21s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=566.67nm - setup: EE 227.3ms, inv.: 1.7ms, repropa.: 2.7ms (1 field configs), tot: 231.8ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=575.00nm - setup: EE 237.9ms, inv.: 1.8ms, repropa.: 2.2ms (1 field configs), tot: 242.0ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=583.33nm - setup: EE 234.9ms, inv.: 1.7ms, repropa.: 2.4ms (1 field configs), tot: 239.0ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=591.67nm - setup: EE 234.5ms, inv.: 1.8ms, repropa.: 2.2ms (1 field configs), tot: 238.6ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=600.00nm - setup: EE 236.8ms, inv.: 1.8ms, repropa.: 3.3ms (1 field configs), tot: 242.0ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=608.33nm - setup: EE 235.5ms, inv.: 1.8ms, repropa.: 2.2ms (1 field configs), tot: 239.6ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=616.67nm - setup: EE 236.7ms, inv.: 2.0ms, repropa.: 2.8ms (1 field configs), tot: 241.6ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.21s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=625.00nm - setup: EE 226.3ms, inv.: 1.8ms, repropa.: 2.2ms (1 field configs), tot: 230.4ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=633.33nm - setup: EE 234.4ms, inv.: 1.7ms, repropa.: 2.4ms (1 field configs), tot: 238.6ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=641.67nm - setup: EE 232.1ms, inv.: 1.9ms, repropa.: 3.5ms (1 field configs), tot: 237.6ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.21s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=650.00nm - setup: EE 228.2ms, inv.: 1.7ms, repropa.: 2.4ms (1 field configs), tot: 232.4ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=658.33nm - setup: EE 234.0ms, inv.: 1.8ms, repropa.: 3.1ms (1 field configs), tot: 239.0ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.21s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=666.67nm - setup: EE 226.9ms, inv.: 1.8ms, repropa.: 2.3ms (1 field configs), tot: 231.1ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=675.00nm - setup: EE 233.6ms, inv.: 1.8ms, repropa.: 2.2ms (1 field configs), tot: 237.8ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.21s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=683.33nm - setup: EE 229.2ms, inv.: 1.7ms, repropa.: 2.4ms (1 field configs), tot: 233.3ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.23s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=691.67nm - setup: EE 241.2ms, inv.: 1.9ms, repropa.: 2.2ms (1 field configs), tot: 245.4ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.21s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=700.00nm - setup: EE 229.8ms, inv.: 1.8ms, repropa.: 2.2ms (1 field configs), tot: 233.9ms
timing for wl=450.00nm - setup: EE 4.3ms, inv.: 1.7ms, repropa.: 2.0ms (1 field configs), tot: 8.1ms
timing for wl=458.33nm - setup: EE 3.6ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 7.0ms
timing for wl=466.67nm - setup: EE 3.5ms, inv.: 1.5ms, repropa.: 2.0ms (1 field configs), tot: 7.0ms
timing for wl=475.00nm - setup: EE 3.5ms, inv.: 1.5ms, repropa.: 2.0ms (1 field configs), tot: 7.0ms
timing for wl=483.33nm - setup: EE 4.1ms, inv.: 1.5ms, repropa.: 2.0ms (1 field configs), tot: 7.6ms
timing for wl=491.67nm - setup: EE 3.7ms, inv.: 1.5ms, repropa.: 1.9ms (1 field configs), tot: 7.3ms
timing for wl=500.00nm - setup: EE 3.5ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 6.9ms
timing for wl=508.33nm - setup: EE 3.6ms, inv.: 1.5ms, repropa.: 1.9ms (1 field configs), tot: 7.1ms
timing for wl=516.67nm - setup: EE 3.6ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 7.0ms
timing for wl=525.00nm - setup: EE 3.7ms, inv.: 1.5ms, repropa.: 2.0ms (1 field configs), tot: 7.2ms
timing for wl=533.33nm - setup: EE 3.5ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 6.9ms
timing for wl=541.67nm - setup: EE 3.5ms, inv.: 1.5ms, repropa.: 1.9ms (1 field configs), tot: 6.9ms
timing for wl=550.00nm - setup: EE 3.6ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 6.9ms
timing for wl=558.33nm - setup: EE 3.5ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 6.9ms
timing for wl=566.67nm - setup: EE 3.5ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 6.9ms
timing for wl=575.00nm - setup: EE 3.4ms, inv.: 1.5ms, repropa.: 1.9ms (1 field configs), tot: 6.8ms
timing for wl=583.33nm - setup: EE 3.6ms, inv.: 1.3ms, repropa.: 2.0ms (1 field configs), tot: 7.1ms
timing for wl=591.67nm - setup: EE 3.4ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 6.8ms
timing for wl=600.00nm - setup: EE 3.3ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 6.7ms
timing for wl=608.33nm - setup: EE 3.5ms, inv.: 1.5ms, repropa.: 1.9ms (1 field configs), tot: 7.0ms
timing for wl=616.67nm - setup: EE 3.5ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 6.9ms
timing for wl=625.00nm - setup: EE 3.5ms, inv.: 1.5ms, repropa.: 1.9ms (1 field configs), tot: 7.0ms
timing for wl=633.33nm - setup: EE 3.5ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 6.8ms
timing for wl=641.67nm - setup: EE 3.7ms, inv.: 1.5ms, repropa.: 1.9ms (1 field configs), tot: 7.1ms
timing for wl=650.00nm - setup: EE 3.5ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 6.9ms
timing for wl=658.33nm - setup: EE 3.6ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 7.1ms
timing for wl=666.67nm - setup: EE 3.6ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 6.9ms
timing for wl=675.00nm - setup: EE 3.5ms, inv.: 1.5ms, repropa.: 1.9ms (1 field configs), tot: 6.9ms
timing for wl=683.33nm - setup: EE 3.4ms, inv.: 1.4ms, repropa.: 1.9ms (1 field configs), tot: 6.8ms
timing for wl=691.67nm - setup: EE 3.6ms, inv.: 1.5ms, repropa.: 1.9ms (1 field configs), tot: 7.0ms
timing for wl=700.00nm - setup: EE 3.6ms, inv.: 1.3ms, repropa.: 2.0ms (1 field configs), tot: 7.0ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.21s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=450.00nm - setup: EE 232.7ms, inv.: 1.9ms, repropa.: 2.3ms (1 field configs), tot: 237.0ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.21s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=458.33nm - setup: EE 227.1ms, inv.: 1.7ms, repropa.: 2.3ms (1 field configs), tot: 231.2ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.21s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=466.67nm - setup: EE 227.5ms, inv.: 1.7ms, repropa.: 2.3ms (1 field configs), tot: 231.7ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.21s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=475.00nm - setup: EE 227.1ms, inv.: 1.9ms, repropa.: 2.3ms (1 field configs), tot: 231.4ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=483.33nm - setup: EE 242.2ms, inv.: 1.7ms, repropa.: 3.3ms (1 field configs), tot: 247.4ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=491.67nm - setup: EE 234.4ms, inv.: 1.8ms, repropa.: 2.4ms (1 field configs), tot: 238.7ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.21s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=500.00nm - setup: EE 226.6ms, inv.: 1.7ms, repropa.: 2.7ms (1 field configs), tot: 231.1ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=508.33nm - setup: EE 236.1ms, inv.: 1.8ms, repropa.: 2.3ms (1 field configs), tot: 240.2ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=516.67nm - setup: EE 231.5ms, inv.: 1.7ms, repropa.: 2.4ms (1 field configs), tot: 235.7ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=525.00nm - setup: EE 235.3ms, inv.: 1.9ms, repropa.: 2.4ms (1 field configs), tot: 239.6ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.21s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=533.33nm - setup: EE 230.8ms, inv.: 1.8ms, repropa.: 2.4ms (1 field configs), tot: 235.1ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=541.67nm - setup: EE 233.5ms, inv.: 1.9ms, repropa.: 2.3ms (1 field configs), tot: 237.8ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.23s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=550.00nm - setup: EE 244.4ms, inv.: 1.9ms, repropa.: 3.2ms (1 field configs), tot: 249.5ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.23s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=558.33nm - setup: EE 242.7ms, inv.: 1.9ms, repropa.: 2.3ms (1 field configs), tot: 246.9ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.23s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=566.67nm - setup: EE 241.2ms, inv.: 1.7ms, repropa.: 2.3ms (1 field configs), tot: 245.3ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=575.00nm - setup: EE 241.1ms, inv.: 2.0ms, repropa.: 3.4ms (1 field configs), tot: 246.5ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.23s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=583.33nm - setup: EE 250.8ms, inv.: 1.7ms, repropa.: 2.3ms (1 field configs), tot: 254.9ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.23s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=591.67nm - setup: EE 243.9ms, inv.: 1.8ms, repropa.: 2.3ms (1 field configs), tot: 248.1ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.22s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=600.00nm - setup: EE 241.6ms, inv.: 1.7ms, repropa.: 2.3ms (1 field configs), tot: 245.7ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.23s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=608.33nm - setup: EE 250.8ms, inv.: 1.8ms, repropa.: 2.4ms (1 field configs), tot: 255.1ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.23s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=616.67nm - setup: EE 247.6ms, inv.: 1.7ms, repropa.: 2.6ms (1 field configs), tot: 251.9ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.23s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=625.00nm - setup: EE 250.1ms, inv.: 1.9ms, repropa.: 3.6ms (1 field configs), tot: 255.7ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.24s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=633.33nm - setup: EE 261.5ms, inv.: 1.8ms, repropa.: 3.2ms (1 field configs), tot: 266.6ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.23s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=641.67nm - setup: EE 248.7ms, inv.: 1.9ms, repropa.: 2.4ms (1 field configs), tot: 253.1ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.23s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=650.00nm - setup: EE 245.5ms, inv.: 1.7ms, repropa.: 2.2ms (1 field configs), tot: 249.7ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.24s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=658.33nm - setup: EE 256.4ms, inv.: 1.8ms, repropa.: 2.4ms (1 field configs), tot: 260.7ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.24s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=666.67nm - setup: EE 260.1ms, inv.: 1.8ms, repropa.: 2.4ms (1 field configs), tot: 264.4ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.25s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=675.00nm - setup: EE 266.0ms, inv.: 1.8ms, repropa.: 2.3ms (1 field configs), tot: 270.2ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.25s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=683.33nm - setup: EE 270.3ms, inv.: 1.8ms, repropa.: 3.4ms (1 field configs), tot: 275.5ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.23s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=691.67nm - setup: EE 252.3ms, inv.: 1.9ms, repropa.: 3.2ms (1 field configs), tot: 257.5ms
identification of identical tensors: 0.00s
sorting: 0.00s
indexing of unique tensors: 0.00s
calculating 803 retarded tensors: 0.24s (working on 12 processes)
reconstruction of coupling matrix: 0.00s
timing for wl=700.00nm - setup: EE 255.7ms, inv.: 1.8ms, repropa.: 2.3ms (1 field configs), tot: 259.9ms
timing for wl=450.00nm - setup: EE 72.4ms, inv.: 31.2ms, repropa.: 12.8ms (1 field configs), tot: 117.2ms
timing for wl=458.33nm - setup: EE 72.0ms, inv.: 24.0ms, repropa.: 12.3ms (1 field configs), tot: 108.4ms
timing for wl=466.67nm - setup: EE 71.1ms, inv.: 26.9ms, repropa.: 12.8ms (1 field configs), tot: 111.1ms
timing for wl=475.00nm - setup: EE 73.3ms, inv.: 24.8ms, repropa.: 12.5ms (1 field configs), tot: 110.7ms
timing for wl=483.33nm - setup: EE 72.8ms, inv.: 24.8ms, repropa.: 12.4ms (1 field configs), tot: 110.0ms
timing for wl=491.67nm - setup: EE 71.1ms, inv.: 27.1ms, repropa.: 13.6ms (1 field configs), tot: 111.9ms
timing for wl=500.00nm - setup: EE 74.6ms, inv.: 25.2ms, repropa.: 12.5ms (1 field configs), tot: 112.5ms
timing for wl=508.33nm - setup: EE 73.2ms, inv.: 25.1ms, repropa.: 12.5ms (1 field configs), tot: 111.0ms
timing for wl=516.67nm - setup: EE 71.2ms, inv.: 25.5ms, repropa.: 12.1ms (1 field configs), tot: 109.1ms
timing for wl=525.00nm - setup: EE 71.9ms, inv.: 24.8ms, repropa.: 12.1ms (1 field configs), tot: 108.9ms
timing for wl=533.33nm - setup: EE 71.8ms, inv.: 25.1ms, repropa.: 12.1ms (1 field configs), tot: 109.2ms
timing for wl=541.67nm - setup: EE 71.9ms, inv.: 25.9ms, repropa.: 12.3ms (1 field configs), tot: 110.2ms
timing for wl=550.00nm - setup: EE 72.2ms, inv.: 24.9ms, repropa.: 12.0ms (1 field configs), tot: 109.5ms
timing for wl=558.33nm - setup: EE 71.2ms, inv.: 24.8ms, repropa.: 12.3ms (1 field configs), tot: 108.5ms
timing for wl=566.67nm - setup: EE 71.3ms, inv.: 24.9ms, repropa.: 12.4ms (1 field configs), tot: 108.6ms
timing for wl=575.00nm - setup: EE 74.0ms, inv.: 24.8ms, repropa.: 12.2ms (1 field configs), tot: 111.1ms
timing for wl=583.33nm - setup: EE 72.4ms, inv.: 24.9ms, repropa.: 12.2ms (1 field configs), tot: 109.7ms
timing for wl=591.67nm - setup: EE 71.8ms, inv.: 24.8ms, repropa.: 12.3ms (1 field configs), tot: 109.0ms
timing for wl=600.00nm - setup: EE 71.7ms, inv.: 25.0ms, repropa.: 12.2ms (1 field configs), tot: 109.1ms
timing for wl=608.33nm - setup: EE 70.4ms, inv.: 24.8ms, repropa.: 12.2ms (1 field configs), tot: 107.6ms
timing for wl=616.67nm - setup: EE 72.2ms, inv.: 25.1ms, repropa.: 12.6ms (1 field configs), tot: 110.2ms
timing for wl=625.00nm - setup: EE 71.8ms, inv.: 25.0ms, repropa.: 12.1ms (1 field configs), tot: 109.1ms
timing for wl=633.33nm - setup: EE 72.2ms, inv.: 24.8ms, repropa.: 12.3ms (1 field configs), tot: 109.5ms
timing for wl=641.67nm - setup: EE 72.0ms, inv.: 24.8ms, repropa.: 12.5ms (1 field configs), tot: 109.5ms
timing for wl=650.00nm - setup: EE 72.3ms, inv.: 25.2ms, repropa.: 12.1ms (1 field configs), tot: 109.7ms
timing for wl=658.33nm - setup: EE 73.3ms, inv.: 25.8ms, repropa.: 12.2ms (1 field configs), tot: 111.4ms
timing for wl=666.67nm - setup: EE 71.2ms, inv.: 25.6ms, repropa.: 12.2ms (1 field configs), tot: 109.2ms
timing for wl=675.00nm - setup: EE 71.6ms, inv.: 24.7ms, repropa.: 12.1ms (1 field configs), tot: 108.5ms
timing for wl=683.33nm - setup: EE 72.0ms, inv.: 25.0ms, repropa.: 12.2ms (1 field configs), tot: 109.3ms
timing for wl=691.67nm - setup: EE 71.4ms, inv.: 24.8ms, repropa.: 12.0ms (1 field configs), tot: 108.3ms
timing for wl=700.00nm - setup: EE 72.0ms, inv.: 25.0ms, repropa.: 12.2ms (1 field configs), tot: 109.3ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.78s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=450.00nm - setup: EE 2476.5ms, inv.: 25.7ms, repropa.: 12.5ms (1 field configs), tot: 2514.8ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.79s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=458.33nm - setup: EE 2488.8ms, inv.: 24.3ms, repropa.: 12.3ms (1 field configs), tot: 2525.7ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.78s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=466.67nm - setup: EE 2477.2ms, inv.: 25.0ms, repropa.: 12.5ms (1 field configs), tot: 2514.8ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.79s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=475.00nm - setup: EE 2487.8ms, inv.: 25.0ms, repropa.: 12.4ms (1 field configs), tot: 2525.3ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.81s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=483.33nm - setup: EE 2507.4ms, inv.: 25.9ms, repropa.: 12.6ms (1 field configs), tot: 2546.1ms
identification of identical tensors: 0.03s
sorting: 0.26s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.79s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=491.67nm - setup: EE 2486.2ms, inv.: 25.4ms, repropa.: 12.3ms (1 field configs), tot: 2524.2ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.81s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=500.00nm - setup: EE 2505.1ms, inv.: 25.1ms, repropa.: 12.1ms (1 field configs), tot: 2542.6ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.04s
calculating 11692 retarded tensors: 1.79s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=508.33nm - setup: EE 2486.6ms, inv.: 25.0ms, repropa.: 12.4ms (1 field configs), tot: 2524.2ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.80s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=516.67nm - setup: EE 2493.5ms, inv.: 25.3ms, repropa.: 12.3ms (1 field configs), tot: 2531.4ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.80s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=525.00nm - setup: EE 2497.5ms, inv.: 25.2ms, repropa.: 12.4ms (1 field configs), tot: 2535.4ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.80s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=533.33nm - setup: EE 2494.5ms, inv.: 25.1ms, repropa.: 12.5ms (1 field configs), tot: 2532.3ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.79s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=541.67nm - setup: EE 2490.1ms, inv.: 24.8ms, repropa.: 12.5ms (1 field configs), tot: 2527.6ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.80s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=550.00nm - setup: EE 2491.4ms, inv.: 25.1ms, repropa.: 12.3ms (1 field configs), tot: 2528.9ms
identification of identical tensors: 0.03s
sorting: 0.26s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.80s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=558.33nm - setup: EE 2511.4ms, inv.: 24.9ms, repropa.: 12.7ms (1 field configs), tot: 2549.1ms
identification of identical tensors: 0.03s
sorting: 0.26s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.87s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=566.67nm - setup: EE 2577.9ms, inv.: 25.1ms, repropa.: 12.2ms (1 field configs), tot: 2615.4ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.79s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=575.00nm - setup: EE 2486.6ms, inv.: 24.9ms, repropa.: 12.5ms (1 field configs), tot: 2524.2ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.80s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=583.33nm - setup: EE 2502.1ms, inv.: 26.1ms, repropa.: 12.4ms (1 field configs), tot: 2540.8ms
identification of identical tensors: 0.03s
sorting: 0.26s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.81s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=591.67nm - setup: EE 2509.9ms, inv.: 25.3ms, repropa.: 12.3ms (1 field configs), tot: 2547.7ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.80s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=600.00nm - setup: EE 2496.1ms, inv.: 25.1ms, repropa.: 12.2ms (1 field configs), tot: 2533.6ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.79s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=608.33nm - setup: EE 2491.7ms, inv.: 25.0ms, repropa.: 12.5ms (1 field configs), tot: 2529.4ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.79s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=616.67nm - setup: EE 2497.5ms, inv.: 24.9ms, repropa.: 12.6ms (1 field configs), tot: 2535.2ms
identification of identical tensors: 0.03s
sorting: 0.27s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.87s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=625.00nm - setup: EE 2594.0ms, inv.: 24.8ms, repropa.: 12.7ms (1 field configs), tot: 2631.7ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.91s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=633.33nm - setup: EE 2615.2ms, inv.: 25.5ms, repropa.: 12.4ms (1 field configs), tot: 2653.2ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.87s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=641.67nm - setup: EE 2577.8ms, inv.: 25.5ms, repropa.: 12.8ms (1 field configs), tot: 2616.2ms
identification of identical tensors: 0.03s
sorting: 0.26s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.87s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=650.00nm - setup: EE 2573.0ms, inv.: 25.9ms, repropa.: 12.6ms (1 field configs), tot: 2611.7ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.84s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=658.33nm - setup: EE 2546.0ms, inv.: 25.4ms, repropa.: 12.5ms (1 field configs), tot: 2584.0ms
identification of identical tensors: 0.03s
sorting: 0.27s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.87s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=666.67nm - setup: EE 2593.0ms, inv.: 27.8ms, repropa.: 12.6ms (1 field configs), tot: 2633.7ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.83s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=675.00nm - setup: EE 2531.8ms, inv.: 24.7ms, repropa.: 12.3ms (1 field configs), tot: 2569.0ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.81s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=683.33nm - setup: EE 2506.5ms, inv.: 27.6ms, repropa.: 12.3ms (1 field configs), tot: 2546.8ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.82s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=691.67nm - setup: EE 2520.9ms, inv.: 25.8ms, repropa.: 12.9ms (1 field configs), tot: 2559.8ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11692 retarded tensors: 1.81s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=700.00nm - setup: EE 2507.8ms, inv.: 25.3ms, repropa.: 12.5ms (1 field configs), tot: 2545.7ms
timing for wl=450.00nm - setup: EE 71.2ms, inv.: 25.5ms, repropa.: 12.4ms (1 field configs), tot: 109.5ms
timing for wl=458.33nm - setup: EE 71.2ms, inv.: 24.1ms, repropa.: 12.4ms (1 field configs), tot: 107.8ms
timing for wl=466.67nm - setup: EE 71.5ms, inv.: 24.9ms, repropa.: 12.5ms (1 field configs), tot: 109.2ms
timing for wl=475.00nm - setup: EE 72.1ms, inv.: 24.8ms, repropa.: 12.5ms (1 field configs), tot: 109.5ms
timing for wl=483.33nm - setup: EE 71.8ms, inv.: 27.1ms, repropa.: 12.5ms (1 field configs), tot: 111.6ms
timing for wl=491.67nm - setup: EE 73.7ms, inv.: 27.4ms, repropa.: 12.4ms (1 field configs), tot: 113.8ms
timing for wl=500.00nm - setup: EE 74.1ms, inv.: 25.1ms, repropa.: 12.4ms (1 field configs), tot: 111.7ms
timing for wl=508.33nm - setup: EE 72.1ms, inv.: 24.8ms, repropa.: 12.4ms (1 field configs), tot: 109.5ms
timing for wl=516.67nm - setup: EE 72.2ms, inv.: 25.0ms, repropa.: 12.5ms (1 field configs), tot: 109.8ms
timing for wl=525.00nm - setup: EE 71.9ms, inv.: 24.9ms, repropa.: 12.4ms (1 field configs), tot: 109.4ms
timing for wl=533.33nm - setup: EE 71.8ms, inv.: 25.1ms, repropa.: 12.8ms (1 field configs), tot: 109.9ms
timing for wl=541.67nm - setup: EE 71.9ms, inv.: 24.8ms, repropa.: 12.5ms (1 field configs), tot: 109.4ms
timing for wl=550.00nm - setup: EE 72.6ms, inv.: 25.0ms, repropa.: 12.5ms (1 field configs), tot: 110.5ms
timing for wl=558.33nm - setup: EE 71.8ms, inv.: 24.7ms, repropa.: 12.7ms (1 field configs), tot: 109.3ms
timing for wl=566.67nm - setup: EE 71.7ms, inv.: 25.0ms, repropa.: 12.6ms (1 field configs), tot: 109.4ms
timing for wl=575.00nm - setup: EE 71.7ms, inv.: 24.9ms, repropa.: 12.9ms (1 field configs), tot: 109.7ms
timing for wl=583.33nm - setup: EE 72.5ms, inv.: 25.0ms, repropa.: 12.6ms (1 field configs), tot: 110.2ms
timing for wl=591.67nm - setup: EE 71.6ms, inv.: 24.8ms, repropa.: 12.3ms (1 field configs), tot: 108.8ms
timing for wl=600.00nm - setup: EE 72.1ms, inv.: 25.9ms, repropa.: 12.7ms (1 field configs), tot: 110.8ms
timing for wl=608.33nm - setup: EE 72.0ms, inv.: 24.9ms, repropa.: 12.6ms (1 field configs), tot: 109.6ms
timing for wl=616.67nm - setup: EE 71.8ms, inv.: 24.8ms, repropa.: 12.6ms (1 field configs), tot: 109.4ms
timing for wl=625.00nm - setup: EE 71.7ms, inv.: 24.8ms, repropa.: 12.5ms (1 field configs), tot: 109.2ms
timing for wl=633.33nm - setup: EE 71.6ms, inv.: 24.8ms, repropa.: 12.7ms (1 field configs), tot: 109.3ms
timing for wl=641.67nm - setup: EE 72.0ms, inv.: 24.8ms, repropa.: 12.5ms (1 field configs), tot: 109.4ms
timing for wl=650.00nm - setup: EE 71.9ms, inv.: 25.0ms, repropa.: 12.5ms (1 field configs), tot: 109.5ms
timing for wl=658.33nm - setup: EE 73.4ms, inv.: 24.8ms, repropa.: 12.5ms (1 field configs), tot: 110.8ms
timing for wl=666.67nm - setup: EE 72.6ms, inv.: 25.0ms, repropa.: 12.4ms (1 field configs), tot: 110.2ms
timing for wl=675.00nm - setup: EE 72.6ms, inv.: 25.2ms, repropa.: 12.3ms (1 field configs), tot: 110.4ms
timing for wl=683.33nm - setup: EE 71.9ms, inv.: 25.0ms, repropa.: 12.5ms (1 field configs), tot: 109.6ms
timing for wl=691.67nm - setup: EE 72.5ms, inv.: 25.0ms, repropa.: 12.5ms (1 field configs), tot: 110.2ms
timing for wl=700.00nm - setup: EE 72.0ms, inv.: 25.2ms, repropa.: 12.4ms (1 field configs), tot: 109.9ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 1.76s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=450.00nm - setup: EE 2463.2ms, inv.: 25.7ms, repropa.: 12.7ms (1 field configs), tot: 2501.9ms
identification of identical tensors: 0.03s
sorting: 0.26s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 1.72s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=458.33nm - setup: EE 2434.5ms, inv.: 27.1ms, repropa.: 13.5ms (1 field configs), tot: 2475.3ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 1.76s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=466.67nm - setup: EE 2452.4ms, inv.: 26.6ms, repropa.: 12.5ms (1 field configs), tot: 2491.8ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 1.86s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=475.00nm - setup: EE 2569.1ms, inv.: 27.2ms, repropa.: 13.3ms (1 field configs), tot: 2609.8ms
identification of identical tensors: 0.03s
sorting: 0.26s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 1.81s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=483.33nm - setup: EE 2527.7ms, inv.: 25.5ms, repropa.: 12.7ms (1 field configs), tot: 2566.2ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 1.79s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=491.67nm - setup: EE 2490.1ms, inv.: 26.2ms, repropa.: 12.4ms (1 field configs), tot: 2528.9ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 1.79s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=500.00nm - setup: EE 2488.2ms, inv.: 24.8ms, repropa.: 12.4ms (1 field configs), tot: 2525.7ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 1.75s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=508.33nm - setup: EE 2446.6ms, inv.: 24.8ms, repropa.: 12.7ms (1 field configs), tot: 2484.3ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 1.84s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=516.67nm - setup: EE 2532.4ms, inv.: 27.3ms, repropa.: 12.9ms (1 field configs), tot: 2572.9ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 1.78s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=525.00nm - setup: EE 2487.3ms, inv.: 25.0ms, repropa.: 12.4ms (1 field configs), tot: 2524.8ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 1.73s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=533.33nm - setup: EE 2429.0ms, inv.: 24.8ms, repropa.: 12.7ms (1 field configs), tot: 2466.6ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 1.81s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=541.67nm - setup: EE 2510.2ms, inv.: 26.7ms, repropa.: 12.6ms (1 field configs), tot: 2549.7ms
identification of identical tensors: 0.03s
sorting: 0.26s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 1.78s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=550.00nm - setup: EE 2494.2ms, inv.: 26.4ms, repropa.: 12.8ms (1 field configs), tot: 2533.7ms
identification of identical tensors: 0.03s
sorting: 0.26s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 2.02s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=558.33nm - setup: EE 2724.5ms, inv.: 26.8ms, repropa.: 12.8ms (1 field configs), tot: 2764.1ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 2.04s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=566.67nm - setup: EE 2748.2ms, inv.: 27.0ms, repropa.: 12.9ms (1 field configs), tot: 2788.2ms
identification of identical tensors: 0.03s
sorting: 0.26s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 2.08s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=575.00nm - setup: EE 2787.0ms, inv.: 26.9ms, repropa.: 13.3ms (1 field configs), tot: 2827.6ms
identification of identical tensors: 0.03s
sorting: 0.26s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 2.09s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=583.33nm - setup: EE 2801.5ms, inv.: 27.5ms, repropa.: 12.4ms (1 field configs), tot: 2841.6ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.04s
calculating 11365 retarded tensors: 2.05s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=591.67nm - setup: EE 2744.7ms, inv.: 26.3ms, repropa.: 12.3ms (1 field configs), tot: 2783.5ms
identification of identical tensors: 0.03s
sorting: 0.27s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 1.96s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=600.00nm - setup: EE 2681.7ms, inv.: 27.1ms, repropa.: 12.7ms (1 field configs), tot: 2721.7ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 2.02s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=608.33nm - setup: EE 2724.8ms, inv.: 27.6ms, repropa.: 12.9ms (1 field configs), tot: 2765.4ms
identification of identical tensors: 0.03s
sorting: 0.26s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 1.95s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=616.67nm - setup: EE 2663.4ms, inv.: 26.7ms, repropa.: 13.5ms (1 field configs), tot: 2703.7ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 1.99s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=625.00nm - setup: EE 2690.9ms, inv.: 26.7ms, repropa.: 12.7ms (1 field configs), tot: 2730.6ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 2.03s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=633.33nm - setup: EE 2727.2ms, inv.: 26.8ms, repropa.: 17.0ms (1 field configs), tot: 2771.1ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 1.99s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=641.67nm - setup: EE 2686.3ms, inv.: 27.4ms, repropa.: 12.4ms (1 field configs), tot: 2726.2ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 2.06s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=650.00nm - setup: EE 2759.1ms, inv.: 27.7ms, repropa.: 12.6ms (1 field configs), tot: 2799.6ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 2.35s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=658.33nm - setup: EE 3054.5ms, inv.: 24.8ms, repropa.: 12.7ms (1 field configs), tot: 3092.3ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 2.25s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=666.67nm - setup: EE 2954.0ms, inv.: 27.6ms, repropa.: 12.3ms (1 field configs), tot: 2994.0ms
identification of identical tensors: 0.03s
sorting: 0.26s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 2.33s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=675.00nm - setup: EE 3049.9ms, inv.: 25.3ms, repropa.: 12.9ms (1 field configs), tot: 3088.2ms
identification of identical tensors: 0.03s
sorting: 0.26s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 2.33s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=683.33nm - setup: EE 3036.0ms, inv.: 24.8ms, repropa.: 12.1ms (1 field configs), tot: 3073.3ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.05s
calculating 11365 retarded tensors: 2.20s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=691.67nm - setup: EE 2895.7ms, inv.: 25.2ms, repropa.: 12.5ms (1 field configs), tot: 2933.6ms
identification of identical tensors: 0.03s
sorting: 0.25s
indexing of unique tensors: 0.04s
calculating 11365 retarded tensors: 2.13s (working on 12 processes)
reconstruction of coupling matrix: 0.02s
timing for wl=700.00nm - setup: EE 2829.8ms, inv.: 26.7ms, repropa.: 12.4ms (1 field configs), tot: 2869.1ms
plot the spectra¶
[4]:
colors = ['C0', 'C0', 'C1', 'C1']
dashes = [[2,2], [], [2,2], []]
## --- plot small sphere results
plt.figure()
for i in range(4): # small sphere configs
plt.plot(wl, all_spec[i], label=all_tit[i], color=colors[i], dashes=dashes[i])
plt.legend(fontsize=9)
plt.xlabel('wavelength (nm)', fontsize=14)
plt.ylabel('extinction section (nm^2)', fontsize=14)
plt.show()
## --- plot large sphere results
plt.figure()
for i in range(4, 8): # large sphere configs
plt.plot(wl, all_spec[i], label=all_tit[i], color=colors[i%4], dashes=dashes[i%4])
plt.legend(fontsize=9)
plt.xlabel('wavelength (nm)', fontsize=14)
plt.ylabel('extinction section (nm^2)', fontsize=14)
plt.show()
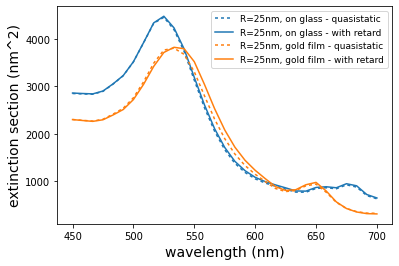
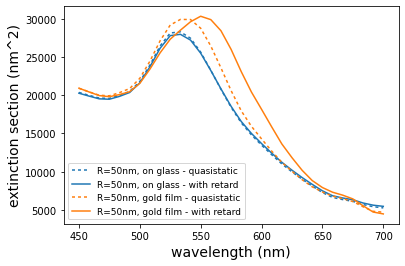