Optical forces¶
!!Attention!!: linear.optical_force is still beta functionality and is to be used with caution.
Author: Clément Majorel (field gradients code also by C. Majorel)
In this example, we calculate the optical force between two nano-cubes, trying to reproduce the physics reported by Chaumet & Nieto-Vesperinas [1].
First we load pyGDM, construct the geometry and setup the simulation.
[1]: Chaumet & Nieto-Vesperinas: Coupled dipole method determination of the electromagnetic force on a particle over a flat dielectric substrate PRB 61, 14119-14127 (2000) (https://journals.aps.org/prb/abstract/10.1103/PhysRevB.61.14119)
[1]:
import numpy as np
import matplotlib.pyplot as plt
from pyGDM2 import fields
from pyGDM2 import tools
from pyGDM2 import structures
from pyGDM2 import propagators
from pyGDM2 import core
from pyGDM2 import linear
from pyGDM2 import visu
from pyGDM2 import materials
## -- global parameters
mesh = 'cube'
step = 33.
## -- simulations for several distances between nano-cubes
gap_list = np.linspace(0, 500, 100)
all_F = []
for gap in gap_list:
## -- create nanostructure of 2 cubes
material = materials.dummy(n=2)
geo1 = structures.rect_wire(step, L=5, H=5, W=5)
geo2 = structures.rect_wire(step, L=5, H=5, W=5)
geo2 = structures.shift(geo2, [0, 0, -1*(5*step+gap)])
geo = structures.combine_geometries([geo1, geo2], step)
struct = structures.struct(step, geo, material, verbose=False)
## -- incident field
field_generator = fields.plane_wave
kwargs = dict(E_p=1, inc_angle=0) ## normal incidence, x-polarization
wavelengths = [633.]
efield = fields.efield(field_generator, wavelengths=wavelengths, kwargs=kwargs)
## -- environment
n1, n2, n3 = 1., 1., 1.
dyads = propagators.DyadsQuasistatic123(n1, n2, n3, spacing=99999)
## -- simulation
sim = core.simulation(struct, efield, dyads)
#visu.structure(sim, show=1, projection='xz')
print("Nr of dipoles: {}; distance: {}nm".format(len(geo), gap))
## -- run simulation
core.scatter(sim, method='cupy')
## -- split one half of structure, calculated fields are kept
sim_part1, sim_part2 = tools.split_simulation(sim, geo1)
sim_subpart = sim_part1
# =============================================================================
# the actual calculation of the force on one of the cubes
# following Eq. (6) from Chaumet & Nieto-Vesperinas, PRB 61, 14119–14127 (2000)
# =============================================================================
field_index = 0
F_tot = linear.optical_force(sim_subpart, field_index)
all_F.append(F_tot)
all_F = np.array(all_F)
Nr of dipoles: 250; distance: 0.0nm
/home/hans/.local/lib/python3.8/site-packages/numba/core/dispatcher.py:237: UserWarning: Numba extension module 'numba_scipy' failed to load due to 'ValueError(No function '__pyx_fuse_0pdtr' found in __pyx_capi__ of 'scipy.special.cython_special')'.
entrypoints.init_all()
timing for wl=633.00nm - setup: EE 10844.1ms, inv.: 572.3ms, repropa.: 15622.4ms (1 field configs), tot: 27039.3ms
/home/hans/.local/lib/python3.8/site-packages/pyGDM2/linear.py:1135: UserWarning: Optical force calculation is a beta-functionality and still under testing. Please use with caution.
warnings.warn("Optical force calculation is a beta-functionality and still under testing. " +
/home/hans/.local/lib/python3.8/site-packages/pyGDM2/linear.py:894: UserWarning: No H-values inside structure calculated. Setting internal magnetic field zero. Please run 'scatter' with the according parameter.
warnings.warn("No H-values inside structure calculated. Setting internal magnetic field zero. " +
Nr of dipoles: 250; distance: 5.05050505050505nm
timing for wl=633.00nm - setup: EE 18.8ms, inv.: 6.6ms, repropa.: 17.5ms (1 field configs), tot: 43.2ms
/home/hans/.local/lib/python3.8/site-packages/pyGDM2/linear.py:1135: UserWarning: Optical force calculation is a beta-functionality and still under testing. Please use with caution.
warnings.warn("Optical force calculation is a beta-functionality and still under testing. " +
Nr of dipoles: 250; distance: 10.1010101010101nm
timing for wl=633.00nm - setup: EE 17.2ms, inv.: 3.8ms, repropa.: 13.7ms (1 field configs), tot: 34.8ms
Nr of dipoles: 250; distance: 15.15151515151515nm
timing for wl=633.00nm - setup: EE 15.9ms, inv.: 3.8ms, repropa.: 12.7ms (1 field configs), tot: 32.8ms
Nr of dipoles: 250; distance: 20.2020202020202nm
timing for wl=633.00nm - setup: EE 17.4ms, inv.: 4.2ms, repropa.: 12.9ms (1 field configs), tot: 34.7ms
Nr of dipoles: 250; distance: 25.252525252525253nm
timing for wl=633.00nm - setup: EE 15.5ms, inv.: 3.9ms, repropa.: 12.6ms (1 field configs), tot: 32.0ms
Nr of dipoles: 250; distance: 30.3030303030303nm
timing for wl=633.00nm - setup: EE 14.8ms, inv.: 4.0ms, repropa.: 13.6ms (1 field configs), tot: 32.6ms
Nr of dipoles: 250; distance: 35.35353535353535nm
timing for wl=633.00nm - setup: EE 14.7ms, inv.: 4.1ms, repropa.: 12.4ms (1 field configs), tot: 31.3ms
Nr of dipoles: 250; distance: 40.4040404040404nm
timing for wl=633.00nm - setup: EE 17.0ms, inv.: 3.7ms, repropa.: 13.1ms (1 field configs), tot: 34.0ms
Nr of dipoles: 250; distance: 45.45454545454545nm
timing for wl=633.00nm - setup: EE 14.7ms, inv.: 3.8ms, repropa.: 12.7ms (1 field configs), tot: 31.3ms
Nr of dipoles: 250; distance: 50.505050505050505nm
timing for wl=633.00nm - setup: EE 17.0ms, inv.: 3.8ms, repropa.: 13.0ms (1 field configs), tot: 34.0ms
Nr of dipoles: 250; distance: 55.55555555555555nm
timing for wl=633.00nm - setup: EE 19.3ms, inv.: 3.8ms, repropa.: 13.6ms (1 field configs), tot: 36.9ms
Nr of dipoles: 250; distance: 60.6060606060606nm
timing for wl=633.00nm - setup: EE 14.5ms, inv.: 4.9ms, repropa.: 14.5ms (1 field configs), tot: 34.0ms
Nr of dipoles: 250; distance: 65.65656565656565nm
timing for wl=633.00nm - setup: EE 15.4ms, inv.: 3.7ms, repropa.: 14.0ms (1 field configs), tot: 33.4ms
Nr of dipoles: 250; distance: 70.7070707070707nm
timing for wl=633.00nm - setup: EE 17.0ms, inv.: 4.3ms, repropa.: 12.8ms (1 field configs), tot: 34.3ms
Nr of dipoles: 250; distance: 75.75757575757575nm
timing for wl=633.00nm - setup: EE 14.5ms, inv.: 4.0ms, repropa.: 12.7ms (1 field configs), tot: 31.4ms
Nr of dipoles: 250; distance: 80.8080808080808nm
timing for wl=633.00nm - setup: EE 16.3ms, inv.: 3.8ms, repropa.: 13.2ms (1 field configs), tot: 33.4ms
Nr of dipoles: 250; distance: 85.85858585858585nm
timing for wl=633.00nm - setup: EE 19.9ms, inv.: 3.8ms, repropa.: 13.4ms (1 field configs), tot: 37.4ms
Nr of dipoles: 250; distance: 90.9090909090909nm
timing for wl=633.00nm - setup: EE 15.1ms, inv.: 3.8ms, repropa.: 13.0ms (1 field configs), tot: 32.2ms
Nr of dipoles: 250; distance: 95.95959595959596nm
timing for wl=633.00nm - setup: EE 16.7ms, inv.: 4.0ms, repropa.: 13.3ms (1 field configs), tot: 34.2ms
Nr of dipoles: 250; distance: 101.01010101010101nm
timing for wl=633.00nm - setup: EE 16.6ms, inv.: 4.2ms, repropa.: 13.0ms (1 field configs), tot: 34.0ms
Nr of dipoles: 250; distance: 106.06060606060605nm
timing for wl=633.00nm - setup: EE 16.4ms, inv.: 3.7ms, repropa.: 12.8ms (1 field configs), tot: 33.3ms
Nr of dipoles: 250; distance: 111.1111111111111nm
timing for wl=633.00nm - setup: EE 15.6ms, inv.: 4.4ms, repropa.: 14.2ms (1 field configs), tot: 34.4ms
Nr of dipoles: 250; distance: 116.16161616161615nm
timing for wl=633.00nm - setup: EE 14.5ms, inv.: 4.4ms, repropa.: 13.3ms (1 field configs), tot: 32.5ms
Nr of dipoles: 250; distance: 121.2121212121212nm
timing for wl=633.00nm - setup: EE 16.8ms, inv.: 3.7ms, repropa.: 13.3ms (1 field configs), tot: 34.2ms
Nr of dipoles: 250; distance: 126.26262626262626nm
timing for wl=633.00nm - setup: EE 16.1ms, inv.: 4.2ms, repropa.: 13.3ms (1 field configs), tot: 33.9ms
Nr of dipoles: 250; distance: 131.3131313131313nm
timing for wl=633.00nm - setup: EE 16.2ms, inv.: 3.7ms, repropa.: 13.0ms (1 field configs), tot: 33.3ms
Nr of dipoles: 250; distance: 136.36363636363635nm
timing for wl=633.00nm - setup: EE 17.0ms, inv.: 3.7ms, repropa.: 13.0ms (1 field configs), tot: 34.1ms
Nr of dipoles: 250; distance: 141.4141414141414nm
timing for wl=633.00nm - setup: EE 19.7ms, inv.: 3.7ms, repropa.: 13.4ms (1 field configs), tot: 36.9ms
Nr of dipoles: 250; distance: 146.46464646464645nm
timing for wl=633.00nm - setup: EE 15.3ms, inv.: 3.7ms, repropa.: 12.9ms (1 field configs), tot: 32.1ms
Nr of dipoles: 250; distance: 151.5151515151515nm
timing for wl=633.00nm - setup: EE 17.0ms, inv.: 3.7ms, repropa.: 13.0ms (1 field configs), tot: 33.8ms
Nr of dipoles: 250; distance: 156.56565656565655nm
timing for wl=633.00nm - setup: EE 17.0ms, inv.: 3.7ms, repropa.: 13.8ms (1 field configs), tot: 34.6ms
Nr of dipoles: 250; distance: 161.6161616161616nm
timing for wl=633.00nm - setup: EE 15.4ms, inv.: 4.1ms, repropa.: 13.7ms (1 field configs), tot: 33.4ms
Nr of dipoles: 250; distance: 166.66666666666666nm
timing for wl=633.00nm - setup: EE 15.6ms, inv.: 3.9ms, repropa.: 13.2ms (1 field configs), tot: 32.9ms
Nr of dipoles: 250; distance: 171.7171717171717nm
timing for wl=633.00nm - setup: EE 17.0ms, inv.: 3.7ms, repropa.: 12.7ms (1 field configs), tot: 33.5ms
Nr of dipoles: 250; distance: 176.76767676767676nm
timing for wl=633.00nm - setup: EE 15.3ms, inv.: 4.1ms, repropa.: 12.6ms (1 field configs), tot: 32.2ms
Nr of dipoles: 250; distance: 181.8181818181818nm
timing for wl=633.00nm - setup: EE 15.9ms, inv.: 3.8ms, repropa.: 12.7ms (1 field configs), tot: 32.6ms
Nr of dipoles: 250; distance: 186.86868686868686nm
timing for wl=633.00nm - setup: EE 17.2ms, inv.: 3.7ms, repropa.: 13.1ms (1 field configs), tot: 34.1ms
Nr of dipoles: 250; distance: 191.91919191919192nm
timing for wl=633.00nm - setup: EE 17.2ms, inv.: 4.2ms, repropa.: 13.1ms (1 field configs), tot: 34.8ms
Nr of dipoles: 250; distance: 196.96969696969697nm
timing for wl=633.00nm - setup: EE 20.2ms, inv.: 4.3ms, repropa.: 15.2ms (1 field configs), tot: 39.9ms
Nr of dipoles: 250; distance: 202.02020202020202nm
timing for wl=633.00nm - setup: EE 15.9ms, inv.: 3.9ms, repropa.: 12.7ms (1 field configs), tot: 32.7ms
Nr of dipoles: 250; distance: 207.07070707070704nm
timing for wl=633.00nm - setup: EE 16.4ms, inv.: 3.8ms, repropa.: 13.3ms (1 field configs), tot: 33.6ms
Nr of dipoles: 250; distance: 212.1212121212121nm
timing for wl=633.00nm - setup: EE 15.8ms, inv.: 3.8ms, repropa.: 13.3ms (1 field configs), tot: 33.4ms
Nr of dipoles: 250; distance: 217.17171717171715nm
timing for wl=633.00nm - setup: EE 16.0ms, inv.: 3.8ms, repropa.: 12.9ms (1 field configs), tot: 32.8ms
Nr of dipoles: 250; distance: 222.2222222222222nm
timing for wl=633.00nm - setup: EE 16.7ms, inv.: 3.7ms, repropa.: 12.7ms (1 field configs), tot: 33.4ms
Nr of dipoles: 250; distance: 227.27272727272725nm
timing for wl=633.00nm - setup: EE 15.0ms, inv.: 3.8ms, repropa.: 12.6ms (1 field configs), tot: 31.5ms
Nr of dipoles: 250; distance: 232.3232323232323nm
timing for wl=633.00nm - setup: EE 15.8ms, inv.: 3.7ms, repropa.: 12.4ms (1 field configs), tot: 32.2ms
Nr of dipoles: 250; distance: 237.37373737373736nm
timing for wl=633.00nm - setup: EE 16.6ms, inv.: 3.7ms, repropa.: 12.6ms (1 field configs), tot: 33.1ms
Nr of dipoles: 250; distance: 242.4242424242424nm
timing for wl=633.00nm - setup: EE 16.6ms, inv.: 3.6ms, repropa.: 12.5ms (1 field configs), tot: 32.9ms
Nr of dipoles: 250; distance: 247.47474747474746nm
timing for wl=633.00nm - setup: EE 15.7ms, inv.: 4.0ms, repropa.: 12.7ms (1 field configs), tot: 32.5ms
Nr of dipoles: 250; distance: 252.5252525252525nm
timing for wl=633.00nm - setup: EE 15.7ms, inv.: 4.0ms, repropa.: 15.1ms (1 field configs), tot: 35.0ms
Nr of dipoles: 250; distance: 257.57575757575756nm
timing for wl=633.00nm - setup: EE 17.1ms, inv.: 3.8ms, repropa.: 13.0ms (1 field configs), tot: 34.0ms
Nr of dipoles: 250; distance: 262.6262626262626nm
timing for wl=633.00nm - setup: EE 15.1ms, inv.: 4.1ms, repropa.: 12.7ms (1 field configs), tot: 32.2ms
Nr of dipoles: 250; distance: 267.67676767676767nm
timing for wl=633.00nm - setup: EE 15.1ms, inv.: 4.1ms, repropa.: 12.7ms (1 field configs), tot: 32.1ms
Nr of dipoles: 250; distance: 272.7272727272727nm
timing for wl=633.00nm - setup: EE 15.2ms, inv.: 3.8ms, repropa.: 12.7ms (1 field configs), tot: 31.9ms
Nr of dipoles: 250; distance: 277.77777777777777nm
timing for wl=633.00nm - setup: EE 16.1ms, inv.: 4.1ms, repropa.: 13.2ms (1 field configs), tot: 33.5ms
Nr of dipoles: 250; distance: 282.8282828282828nm
timing for wl=633.00nm - setup: EE 14.8ms, inv.: 3.8ms, repropa.: 14.3ms (1 field configs), tot: 33.3ms
Nr of dipoles: 250; distance: 287.8787878787879nm
timing for wl=633.00nm - setup: EE 16.3ms, inv.: 3.8ms, repropa.: 13.3ms (1 field configs), tot: 33.5ms
Nr of dipoles: 250; distance: 292.9292929292929nm
timing for wl=633.00nm - setup: EE 15.4ms, inv.: 4.1ms, repropa.: 13.0ms (1 field configs), tot: 32.5ms
Nr of dipoles: 250; distance: 297.979797979798nm
timing for wl=633.00nm - setup: EE 22.2ms, inv.: 3.9ms, repropa.: 13.0ms (1 field configs), tot: 39.3ms
Nr of dipoles: 250; distance: 303.030303030303nm
timing for wl=633.00nm - setup: EE 17.8ms, inv.: 3.7ms, repropa.: 13.1ms (1 field configs), tot: 34.8ms
Nr of dipoles: 250; distance: 308.0808080808081nm
timing for wl=633.00nm - setup: EE 18.1ms, inv.: 3.8ms, repropa.: 13.3ms (1 field configs), tot: 35.4ms
Nr of dipoles: 250; distance: 313.1313131313131nm
timing for wl=633.00nm - setup: EE 19.5ms, inv.: 4.8ms, repropa.: 13.8ms (1 field configs), tot: 38.3ms
Nr of dipoles: 250; distance: 318.1818181818182nm
timing for wl=633.00nm - setup: EE 17.3ms, inv.: 4.2ms, repropa.: 13.7ms (1 field configs), tot: 35.4ms
Nr of dipoles: 250; distance: 323.2323232323232nm
timing for wl=633.00nm - setup: EE 15.2ms, inv.: 3.9ms, repropa.: 12.7ms (1 field configs), tot: 32.2ms
Nr of dipoles: 250; distance: 328.28282828282823nm
timing for wl=633.00nm - setup: EE 14.3ms, inv.: 4.3ms, repropa.: 12.6ms (1 field configs), tot: 31.7ms
Nr of dipoles: 250; distance: 333.3333333333333nm
timing for wl=633.00nm - setup: EE 15.9ms, inv.: 4.1ms, repropa.: 12.7ms (1 field configs), tot: 32.9ms
Nr of dipoles: 250; distance: 338.38383838383834nm
timing for wl=633.00nm - setup: EE 17.7ms, inv.: 4.0ms, repropa.: 13.7ms (1 field configs), tot: 35.8ms
Nr of dipoles: 250; distance: 343.4343434343434nm
timing for wl=633.00nm - setup: EE 17.5ms, inv.: 4.0ms, repropa.: 16.6ms (1 field configs), tot: 38.3ms
Nr of dipoles: 250; distance: 348.48484848484844nm
timing for wl=633.00nm - setup: EE 16.1ms, inv.: 3.7ms, repropa.: 13.5ms (1 field configs), tot: 33.6ms
Nr of dipoles: 250; distance: 353.5353535353535nm
timing for wl=633.00nm - setup: EE 16.6ms, inv.: 3.9ms, repropa.: 12.6ms (1 field configs), tot: 33.4ms
Nr of dipoles: 250; distance: 358.58585858585855nm
timing for wl=633.00nm - setup: EE 15.8ms, inv.: 3.7ms, repropa.: 14.4ms (1 field configs), tot: 34.0ms
Nr of dipoles: 250; distance: 363.6363636363636nm
timing for wl=633.00nm - setup: EE 21.1ms, inv.: 3.7ms, repropa.: 12.6ms (1 field configs), tot: 37.6ms
Nr of dipoles: 250; distance: 368.68686868686865nm
timing for wl=633.00nm - setup: EE 17.0ms, inv.: 3.8ms, repropa.: 13.1ms (1 field configs), tot: 34.2ms
Nr of dipoles: 250; distance: 373.73737373737373nm
timing for wl=633.00nm - setup: EE 18.2ms, inv.: 4.1ms, repropa.: 13.2ms (1 field configs), tot: 35.8ms
Nr of dipoles: 250; distance: 378.78787878787875nm
timing for wl=633.00nm - setup: EE 18.1ms, inv.: 3.8ms, repropa.: 13.3ms (1 field configs), tot: 35.3ms
Nr of dipoles: 250; distance: 383.83838383838383nm
timing for wl=633.00nm - setup: EE 14.9ms, inv.: 3.9ms, repropa.: 12.5ms (1 field configs), tot: 31.5ms
Nr of dipoles: 250; distance: 388.88888888888886nm
timing for wl=633.00nm - setup: EE 15.2ms, inv.: 3.8ms, repropa.: 13.8ms (1 field configs), tot: 33.0ms
Nr of dipoles: 250; distance: 393.93939393939394nm
timing for wl=633.00nm - setup: EE 15.9ms, inv.: 3.9ms, repropa.: 12.6ms (1 field configs), tot: 32.6ms
Nr of dipoles: 250; distance: 398.98989898989896nm
timing for wl=633.00nm - setup: EE 15.5ms, inv.: 3.8ms, repropa.: 12.7ms (1 field configs), tot: 32.2ms
Nr of dipoles: 250; distance: 404.04040404040404nm
timing for wl=633.00nm - setup: EE 16.3ms, inv.: 3.9ms, repropa.: 12.4ms (1 field configs), tot: 32.8ms
Nr of dipoles: 250; distance: 409.09090909090907nm
timing for wl=633.00nm - setup: EE 15.8ms, inv.: 3.7ms, repropa.: 12.9ms (1 field configs), tot: 32.7ms
Nr of dipoles: 250; distance: 414.1414141414141nm
timing for wl=633.00nm - setup: EE 17.7ms, inv.: 3.8ms, repropa.: 12.7ms (1 field configs), tot: 34.4ms
Nr of dipoles: 250; distance: 419.19191919191917nm
timing for wl=633.00nm - setup: EE 16.1ms, inv.: 3.7ms, repropa.: 13.2ms (1 field configs), tot: 33.1ms
Nr of dipoles: 250; distance: 424.2424242424242nm
timing for wl=633.00nm - setup: EE 16.3ms, inv.: 3.7ms, repropa.: 13.0ms (1 field configs), tot: 33.2ms
Nr of dipoles: 250; distance: 429.2929292929293nm
timing for wl=633.00nm - setup: EE 17.6ms, inv.: 3.8ms, repropa.: 12.8ms (1 field configs), tot: 34.5ms
Nr of dipoles: 250; distance: 434.3434343434343nm
timing for wl=633.00nm - setup: EE 15.5ms, inv.: 3.7ms, repropa.: 12.4ms (1 field configs), tot: 31.7ms
Nr of dipoles: 250; distance: 439.3939393939394nm
timing for wl=633.00nm - setup: EE 15.0ms, inv.: 4.0ms, repropa.: 12.6ms (1 field configs), tot: 31.8ms
Nr of dipoles: 250; distance: 444.4444444444444nm
timing for wl=633.00nm - setup: EE 20.7ms, inv.: 3.9ms, repropa.: 12.8ms (1 field configs), tot: 37.6ms
Nr of dipoles: 250; distance: 449.4949494949495nm
timing for wl=633.00nm - setup: EE 23.7ms, inv.: 3.9ms, repropa.: 14.0ms (1 field configs), tot: 41.8ms
Nr of dipoles: 250; distance: 454.5454545454545nm
timing for wl=633.00nm - setup: EE 17.0ms, inv.: 4.1ms, repropa.: 13.0ms (1 field configs), tot: 34.3ms
Nr of dipoles: 250; distance: 459.5959595959596nm
timing for wl=633.00nm - setup: EE 16.6ms, inv.: 3.8ms, repropa.: 12.5ms (1 field configs), tot: 33.0ms
Nr of dipoles: 250; distance: 464.6464646464646nm
timing for wl=633.00nm - setup: EE 19.5ms, inv.: 3.8ms, repropa.: 13.1ms (1 field configs), tot: 36.5ms
Nr of dipoles: 250; distance: 469.6969696969697nm
timing for wl=633.00nm - setup: EE 16.6ms, inv.: 3.7ms, repropa.: 12.2ms (1 field configs), tot: 32.8ms
Nr of dipoles: 250; distance: 474.7474747474747nm
timing for wl=633.00nm - setup: EE 15.4ms, inv.: 3.8ms, repropa.: 12.4ms (1 field configs), tot: 31.7ms
Nr of dipoles: 250; distance: 479.7979797979798nm
timing for wl=633.00nm - setup: EE 16.9ms, inv.: 4.2ms, repropa.: 12.5ms (1 field configs), tot: 33.7ms
Nr of dipoles: 250; distance: 484.8484848484848nm
timing for wl=633.00nm - setup: EE 16.0ms, inv.: 3.8ms, repropa.: 13.0ms (1 field configs), tot: 33.0ms
Nr of dipoles: 250; distance: 489.89898989898984nm
timing for wl=633.00nm - setup: EE 18.2ms, inv.: 3.7ms, repropa.: 13.5ms (1 field configs), tot: 35.6ms
Nr of dipoles: 250; distance: 494.9494949494949nm
timing for wl=633.00nm - setup: EE 14.6ms, inv.: 4.0ms, repropa.: 13.3ms (1 field configs), tot: 32.2ms
Nr of dipoles: 250; distance: 500.0nm
timing for wl=633.00nm - setup: EE 17.4ms, inv.: 3.7ms, repropa.: 12.8ms (1 field configs), tot: 34.0ms
plot the optical force¶
Now we plot the z-component of the force as function of the inter-particle distance. Note that the relative behvior of the force is the same as in reference [1].
[2]:
plt.plot(gap_list, all_F.T[2], label='z')
plt.legend()
plt.xlabel('distance between nano-cubes (nm)')
plt.ylabel('Fz (a.u.)')
plt.show()
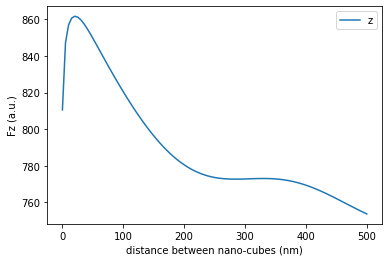