Optical chirality #3¶
03/2021: updated to pyGDM v1.1+
In this example, we reproduce the near-field chirality close to a asymmetric silver from Meinzer et al. [1].
[1]: Meinzer et al.: Probing the chiral nature of electromagnetic fields surrounding plasmonic nanostructures Phys. Rev. B 88(4), 041407 (2013) (https://doi.org/10.1103/PhysRevB.88.041407)
[1]:
from pyGDM2 import structures
from pyGDM2 import materials
from pyGDM2 import fields
from pyGDM2 import core
from pyGDM2 import tools
from pyGDM2 import linear
from pyGDM2 import propagators
from pyGDM2 import visu
import numpy as np
import matplotlib.pyplot as plt
## --- Setup geometries of single rods
mesh = 'cube'
step = 10
W = 160.
L = 60.
H = 30
L_step = int(L/step)
W_step = int(W/step)
H_step = int(H/step)
geometry1 = structures.rect_wire(step, L=L_step, W=W_step, H=H_step, mesh=mesh, ORIENTATION=2)
geometry2 = structures.rect_wire(step, L=L_step, W=W_step, H=H_step, mesh=mesh, ORIENTATION=2)
geometry3 = structures.rect_wire(step, L=L_step, W=W_step, H=H_step, mesh=mesh, ORIENTATION=2)
geometry2.T[0] += 110
geometry3.T[0] += 110
geometry2.T[1] += 80
geometry3.T[1] -= 80
material = materials.silver()
struct1 = structures.struct(step, geometry1, material)
struct2 = structures.struct(step, geometry2, material)
struct3 = structures.struct(step, geometry3, material)
## --- Setup incident field
field_generator = fields.plane_wave
kwargs = dict(inc_angle=180, E_s=1, E_p=0) # from bottom: inc_angle=0, from top: 180
wavelengths = np.linspace(500,1000, 31)
efield = fields.efield(field_generator, wavelengths=wavelengths, kwargs=kwargs)
## --- environment: vacuum
n1, n2, n3 = 1.0, 1.0, 1.0
spacing = 300
dyads = propagators.DyadsQuasistatic123(n1, n2, n3, spacing)
## setup 3 simulations, for the fixed and for the moving blocks
sim1 = core.simulation(struct1, efield, dyads)
sim2 = core.simulation(struct2, efield, dyads)
sim3 = core.simulation(struct3, efield, dyads)
## combine the blocks for L-handed and R-handed dimer
c_sim_L = tools.combine_simulations([sim1, sim2])
c_sim_L = structures.center_struct(c_sim_L)
c_sim_R = tools.combine_simulations([sim1, sim3])
c_sim_R = structures.center_struct(c_sim_R)
visu.structure(c_sim_L)
visu.structure(c_sim_R)
structure initialization - automatic mesh detection: cube
structure initialization - consistency check: 288/288 dipoles valid
structure initialization - automatic mesh detection: cube
structure initialization - consistency check: 288/288 dipoles valid
structure initialization - automatic mesh detection: cube
structure initialization - consistency check: 288/288 dipoles valid
/home/hans/.local/lib/python3.8/site-packages/pyGDM2/visu.py:49: UserWarning: 3D data. Falling back to XY projection...
warnings.warn("3D data. Falling back to XY projection...")
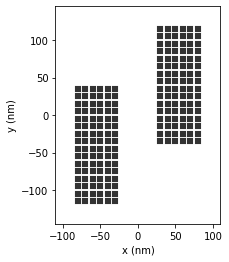
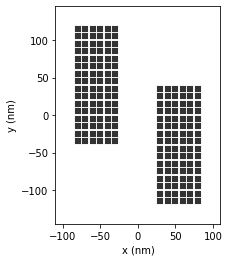
[1]:
<matplotlib.collections.PathCollection at 0x7f743f722070>
running the simulation¶
Now we run the simulations for the left and the right handed dimer.
[2]:
#==============================================================================
# run the simulations
#==============================================================================
core.scatter(c_sim_L, verbose=True)
core.scatter(c_sim_R, verbose=True)
timing for wl=500.00nm - setup: EE 1524.7ms, inv.: 163.6ms, repropa.: 458.8ms (1 field configs), tot: 2147.2ms
timing for wl=516.67nm - setup: EE 27.8ms, inv.: 103.4ms, repropa.: 20.8ms (1 field configs), tot: 152.3ms
timing for wl=533.33nm - setup: EE 30.8ms, inv.: 118.9ms, repropa.: 20.5ms (1 field configs), tot: 170.4ms
timing for wl=550.00nm - setup: EE 29.8ms, inv.: 130.1ms, repropa.: 20.8ms (1 field configs), tot: 180.9ms
timing for wl=566.67nm - setup: EE 29.7ms, inv.: 107.9ms, repropa.: 20.9ms (1 field configs), tot: 158.6ms
timing for wl=583.33nm - setup: EE 29.7ms, inv.: 140.8ms, repropa.: 20.8ms (1 field configs), tot: 191.5ms
timing for wl=600.00nm - setup: EE 32.8ms, inv.: 113.1ms, repropa.: 20.3ms (1 field configs), tot: 166.4ms
timing for wl=616.67nm - setup: EE 33.0ms, inv.: 149.5ms, repropa.: 20.3ms (1 field configs), tot: 203.0ms
timing for wl=633.33nm - setup: EE 31.8ms, inv.: 141.7ms, repropa.: 19.9ms (1 field configs), tot: 193.5ms
timing for wl=650.00nm - setup: EE 32.4ms, inv.: 133.5ms, repropa.: 20.0ms (1 field configs), tot: 186.1ms
timing for wl=666.67nm - setup: EE 31.7ms, inv.: 167.8ms, repropa.: 20.8ms (1 field configs), tot: 220.5ms
timing for wl=683.33nm - setup: EE 33.5ms, inv.: 119.3ms, repropa.: 20.7ms (1 field configs), tot: 173.7ms
timing for wl=700.00nm - setup: EE 32.2ms, inv.: 129.2ms, repropa.: 20.0ms (1 field configs), tot: 181.5ms
timing for wl=716.67nm - setup: EE 30.8ms, inv.: 151.5ms, repropa.: 20.0ms (1 field configs), tot: 202.5ms
timing for wl=733.33nm - setup: EE 31.8ms, inv.: 100.4ms, repropa.: 20.2ms (1 field configs), tot: 153.2ms
timing for wl=750.00nm - setup: EE 30.7ms, inv.: 120.8ms, repropa.: 20.0ms (1 field configs), tot: 171.6ms
timing for wl=766.67nm - setup: EE 30.0ms, inv.: 148.1ms, repropa.: 19.9ms (1 field configs), tot: 198.3ms
timing for wl=783.33nm - setup: EE 29.2ms, inv.: 138.3ms, repropa.: 21.4ms (1 field configs), tot: 189.0ms
timing for wl=800.00nm - setup: EE 31.1ms, inv.: 102.0ms, repropa.: 20.1ms (1 field configs), tot: 153.5ms
timing for wl=816.67nm - setup: EE 29.3ms, inv.: 133.9ms, repropa.: 20.3ms (1 field configs), tot: 184.0ms
timing for wl=833.33nm - setup: EE 29.1ms, inv.: 104.4ms, repropa.: 21.2ms (1 field configs), tot: 154.9ms
timing for wl=850.00nm - setup: EE 30.9ms, inv.: 126.9ms, repropa.: 20.3ms (1 field configs), tot: 178.5ms
timing for wl=866.67nm - setup: EE 33.1ms, inv.: 136.4ms, repropa.: 20.1ms (1 field configs), tot: 189.8ms
timing for wl=883.33nm - setup: EE 31.9ms, inv.: 115.3ms, repropa.: 20.1ms (1 field configs), tot: 167.4ms
timing for wl=900.00nm - setup: EE 32.7ms, inv.: 110.9ms, repropa.: 20.1ms (1 field configs), tot: 165.3ms
timing for wl=916.67nm - setup: EE 39.1ms, inv.: 148.0ms, repropa.: 20.1ms (1 field configs), tot: 207.5ms
timing for wl=933.33nm - setup: EE 31.8ms, inv.: 110.3ms, repropa.: 20.3ms (1 field configs), tot: 162.5ms
timing for wl=950.00nm - setup: EE 32.7ms, inv.: 131.7ms, repropa.: 20.2ms (1 field configs), tot: 186.3ms
timing for wl=966.67nm - setup: EE 31.5ms, inv.: 145.0ms, repropa.: 20.9ms (1 field configs), tot: 197.6ms
timing for wl=983.33nm - setup: EE 32.1ms, inv.: 104.5ms, repropa.: 20.3ms (1 field configs), tot: 157.0ms
timing for wl=1000.00nm - setup: EE 29.6ms, inv.: 114.8ms, repropa.: 20.4ms (1 field configs), tot: 166.2ms
timing for wl=500.00nm - setup: EE 29.6ms, inv.: 117.4ms, repropa.: 20.2ms (1 field configs), tot: 167.5ms
timing for wl=516.67nm - setup: EE 31.5ms, inv.: 141.8ms, repropa.: 20.4ms (1 field configs), tot: 193.8ms
timing for wl=533.33nm - setup: EE 30.4ms, inv.: 142.9ms, repropa.: 23.2ms (1 field configs), tot: 196.6ms
timing for wl=550.00nm - setup: EE 33.6ms, inv.: 146.3ms, repropa.: 20.1ms (1 field configs), tot: 200.8ms
timing for wl=566.67nm - setup: EE 31.4ms, inv.: 106.5ms, repropa.: 20.3ms (1 field configs), tot: 159.6ms
timing for wl=583.33nm - setup: EE 28.4ms, inv.: 153.9ms, repropa.: 20.3ms (1 field configs), tot: 202.8ms
timing for wl=600.00nm - setup: EE 32.2ms, inv.: 99.8ms, repropa.: 20.4ms (1 field configs), tot: 152.6ms
timing for wl=616.67nm - setup: EE 31.1ms, inv.: 108.3ms, repropa.: 20.4ms (1 field configs), tot: 160.4ms
timing for wl=633.33nm - setup: EE 29.8ms, inv.: 170.2ms, repropa.: 20.3ms (1 field configs), tot: 220.4ms
timing for wl=650.00nm - setup: EE 30.1ms, inv.: 157.7ms, repropa.: 20.4ms (1 field configs), tot: 208.4ms
timing for wl=666.67nm - setup: EE 30.5ms, inv.: 141.4ms, repropa.: 22.2ms (1 field configs), tot: 194.7ms
timing for wl=683.33nm - setup: EE 32.7ms, inv.: 101.1ms, repropa.: 20.3ms (1 field configs), tot: 156.4ms
timing for wl=700.00nm - setup: EE 30.1ms, inv.: 127.4ms, repropa.: 20.3ms (1 field configs), tot: 178.1ms
timing for wl=716.67nm - setup: EE 31.7ms, inv.: 100.2ms, repropa.: 20.2ms (1 field configs), tot: 152.3ms
timing for wl=733.33nm - setup: EE 31.9ms, inv.: 122.1ms, repropa.: 20.4ms (1 field configs), tot: 174.6ms
timing for wl=750.00nm - setup: EE 33.8ms, inv.: 140.2ms, repropa.: 21.3ms (1 field configs), tot: 195.5ms
timing for wl=766.67nm - setup: EE 30.6ms, inv.: 107.2ms, repropa.: 20.3ms (1 field configs), tot: 159.7ms
timing for wl=783.33nm - setup: EE 30.4ms, inv.: 119.4ms, repropa.: 20.1ms (1 field configs), tot: 170.1ms
timing for wl=800.00nm - setup: EE 29.6ms, inv.: 134.8ms, repropa.: 20.1ms (1 field configs), tot: 184.6ms
timing for wl=816.67nm - setup: EE 31.1ms, inv.: 131.0ms, repropa.: 20.8ms (1 field configs), tot: 184.4ms
timing for wl=833.33nm - setup: EE 32.7ms, inv.: 102.7ms, repropa.: 20.2ms (1 field configs), tot: 155.8ms
timing for wl=850.00nm - setup: EE 33.6ms, inv.: 161.4ms, repropa.: 20.3ms (1 field configs), tot: 215.5ms
timing for wl=866.67nm - setup: EE 30.8ms, inv.: 101.6ms, repropa.: 20.5ms (1 field configs), tot: 154.6ms
timing for wl=883.33nm - setup: EE 29.3ms, inv.: 136.8ms, repropa.: 20.3ms (1 field configs), tot: 186.5ms
timing for wl=900.00nm - setup: EE 31.8ms, inv.: 163.3ms, repropa.: 20.2ms (1 field configs), tot: 215.4ms
timing for wl=916.67nm - setup: EE 31.0ms, inv.: 99.2ms, repropa.: 20.3ms (1 field configs), tot: 152.2ms
timing for wl=933.33nm - setup: EE 28.6ms, inv.: 126.4ms, repropa.: 20.4ms (1 field configs), tot: 175.6ms
timing for wl=950.00nm - setup: EE 30.7ms, inv.: 147.5ms, repropa.: 20.3ms (1 field configs), tot: 198.7ms
timing for wl=966.67nm - setup: EE 31.1ms, inv.: 99.8ms, repropa.: 20.8ms (1 field configs), tot: 153.2ms
timing for wl=983.33nm - setup: EE 30.4ms, inv.: 121.0ms, repropa.: 20.6ms (1 field configs), tot: 172.1ms
timing for wl=1000.00nm - setup: EE 31.6ms, inv.: 136.6ms, repropa.: 20.6ms (1 field configs), tot: 189.1ms
[2]:
1
Plot the nano-sphere results¶
[3]:
## --- calculate the C spectrum at top of the center between rods
Z_probe = c_sim_L.struct.geometry.T[2].max() + step/2 + 15
## L-dimer
wl, Cspec = tools.calculate_spectrum(c_sim_L, 0, linear.optical_chirality, r_probe=[[0,0,Z_probe]])
C_spec_R = Cspec.T
## R-dimer
wl, Cspec = tools.calculate_spectrum(c_sim_R, 0, linear.optical_chirality, r_probe=[[0,0,Z_probe]])
C_spec_L = Cspec.T
## --- C-mappings at peak (~770nm)
fieldindex = tools.get_closest_field_index(c_sim_R, dict(wavelength=770))
r_probe = tools.generate_NF_map(-150,+150,51, -150,150,51, Z0=Z_probe)
C_R = linear.optical_chirality(c_sim_R, fieldindex, r_probe, which_field='t')
C_L = linear.optical_chirality(c_sim_L, fieldindex, r_probe, which_field='t')
## --- plot
plt.figure(figsize=(9,6))
plt.subplot2grid((3,3), (0,0), aspect='equal')
plt.title("L-dimer")
im = visu.scalarfield(C_L, cmap='jet', show=0)
plt.colorbar(im, label='C / C_LCP')
im.set_clim(-20, 20)
visu.structure_contour(c_sim_L, color='k', dashes=[2,2], show=0)
plt.xticks([]); plt.yticks([])
plt.subplot2grid((3,3), (0,2), aspect='equal')
plt.title("R-dimer")
im = visu.scalarfield(C_R, cmap='jet', show=0)
plt.colorbar(im, label='C / C_LCP')
im.set_clim(-20, 20)
visu.structure_contour(c_sim_R, color='k', dashes=[2,2], show=0)
plt.xticks([]); plt.yticks([])
plt.subplot2grid((3,1), (1,0), rowspan=2, aspect='auto')
# plt.title("R-dimer")
plt.plot(wl, C_spec_R, color='k', label='L-struct')
plt.plot(wl, C_spec_L, color='g', dashes=[2,2], label='R-struct')
plt.xlabel("wavelengh (nm)")
plt.ylabel("C / C_LPC")
plt.tight_layout()
plt.show()
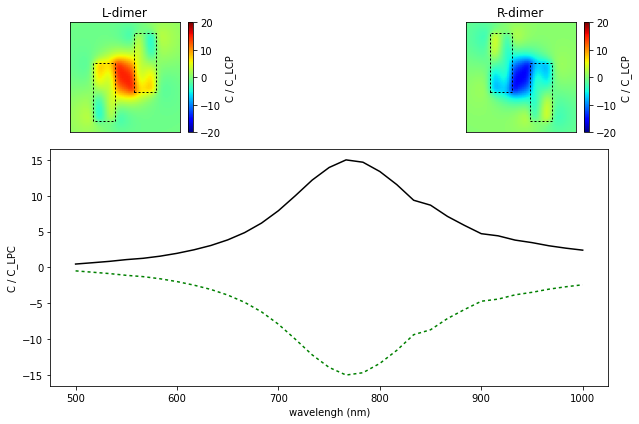